OneSpan Sign Developers: OAuth Event Notification for Salesforce – Part 3
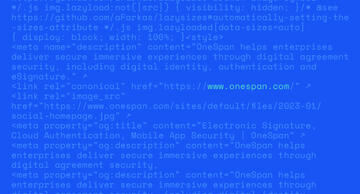
In the last two developer blogs, we walked through basic aspects of registering a connected app in Salesforce with OAuth settings enabled in Part 1 and then focused on managing a refresh token and update OneSpan Sign callback service in Part 2.
This third installment in our ongoing blog series will include a demo project showing how to implement a web service in Salesforce that functions as a callback listener. We’ll break the code into sections and test the entire workflow from transaction creation to receiving and processing notifications.
Prerequisites
To complete these procedures, make sure you have read the previous blogs in the series and completed the following tasks:
- Register a Salesforce Connected App and enable Oauth2 authentication
- Retrieve a refresh token for this app
- Update OneSpan Sign Callback Service
Additionally, you will need to:
- Download the sample code in this Code Share
- (optional) Install OneSpan Sign APEX SDK and become familiar with its basic usage.
Set Up a Callback Listener
In this section, we will break down the sample code section by section. Follow the explanations below and adjust the code accordingly.
Essentially, we exposed an Apex class as a REST web service. The class definition looks like:
@RestResource(urlMapping='/oss/callback') global with sharing class OssCallbackResource{ @HttpPost global static void callback() { …… }
We exposed a web service handling HTTP Post requests at “https://yourdomain.salesforce.com/services/apexrest/oss/callback”. This URL is also known as the callback URL.
Once a OneSpan Sign callback hits our method, the first thing is to parse the inbound JSON payload and to convert it to our own modelling.
OssCallback ossCallback = (OssCallback)JSON.deserialize(RestContext.request.requestbody.tostring(),OssCallback.class); System.debug('Received callback: ' + RestContext.request.requestbody.tostring());
An example inbound payload appears as such:
{ "@class": "com.silanis.esl.packages.event.ESLProcessEvent", "name": "PACKAGE_COMPLETE", "sessionUser": "0787be84-f095-44c7-ba00-787093df86fc", "packageId": "KHiRfOXgKK0gpVWwwpFOSNy6o34=", "message": null, "documentId": null }
If the event name equals “PACKAGE_COMPLETE”, the transaction has been completed, you can handle it as per your business logic:
if(ossCallback.name.equals('PACKAGE_COMPLETE')){ downloadSignedDocuments(ossCallback); }
In our case, we would download all signed documents and the evidence summary to a document folder named “onespan”. Make sure you’ve created this folder, or you can simply adjust the code and store the files anywhere you see fit.
Here we utilized OneSpan APEX SDK functions to facilitate the integration. If you have not installed the APEX SDK or if you are not familiar with its configuration or basic usage, refer to this guide.
private static void downloadSignedDocuments(OssCallback ossCallback){ OneSpanSDK sdk= new OneSpanSDK(); OneSpanAPIObjects.Package_x pack = sdk.getPackage(ossCallback.packageId); List<Document> docs = new List<Document>(); Folder folder = [select id from Folder where name='onespan' LIMIT 1]; for(OneSpanAPIObjects.Document document: pack.documents){ Blob response = sdk.downloadDocument(ossCallback.packageId, document.id); Document doc = new Document(); doc.Body = response; doc.FolderId = folder.id; doc.Name = pack.name + ' - ' + document.name; doc.Type = 'pdf'; docs.add(doc); } //download evidence summary String auditString= sdk.getAudit(ossCallback.packageId); Document auditDoc= new Document(); auditDoc.Body = EncodingUtil.base64Decode(auditString); auditDoc.FolderId = folder.id; auditDoc.Name = pack.name + ' - evidence summary'; auditDoc.Type = 'pdf'; for(Document doc: docs){ insert doc; System.debug('Successfully download file: ' + doc.Name); } insert auditDoc; } }
That’s it! You are now ready to deploy to your own Salesforce environment.
Test the Code
Before our final test, update OneSpan Sign Callback Service and make sure:
- your callback URL is correctly configured
- you’ve at least fired the “PACKAGE_COMPLETE” event
In order to verify if our callback listener works as expected, we can simply create a transaction from sender portal or from any integration points as long as you’ve carried “data” > “origin” : “OSS” in the transaction JSON:
Before the next step, open the Salesforce developer console (In Salesforce > Click your name or the gear icon> Click Developer Console) and monitor the inbound calls in real time.
Now you can finish signing this transaction. In your Salesforce developer console, you will notice that new log(s) come in almost at the same time. Double click on one of them and search “DEBUG”. This filters the logs and only displays the debug level messages we printed with System.debug() function.
Navigate to your Salesforce document folder, and you’ll find the signed documents and the evidence summary there.
This concludes today’s blog. By now, you should be able to implement and deploy a callback listener in Salesforce, properly configure the OneSpan Sign callback service, and test the code.
If you have any questions regarding this blog or anything else concerning the integration of OneSpan Sign into your application, visit the Developer Community Forums. Your feedback matters to us!